티스토리 뷰
이벤트
이벤트가 발생했을 때 호출될 함수를 이벤트 핸들러(event handler)라고 한다.
이벤트가 발생했을 때 브라우저에게 이벤트 핸들러의 호출을 위임하는 것을 이벤트 핸들러 등록 이라한다.
이벤트 핸들러 등록
어트리뷰트 방식
<button onclick="sayHi('Lee')">Click me!</button>
<script>
function sayHi(name){
console.log('Hi! ${name}.');
}
</script>
프로퍼티 방식
<button>Click me!</button>
<script>
// 제이쿼리로 요소 가져오기
const $button = $('button');
// 이벤트 핸들러 프로퍼티에 이벤트 핸들러를 바인딩
$button.onclick = function(){
console.log('button click');
};
</script>
addEventListener 메소드 방식
addEventListener 메소드 방식은 이벤트 핸들러 프로퍼티에 바인딩된 이벤트 핸들러에 아무런 영향을 주지 않는다.
<button>Click me!</button>
<script>
const $button = document.querySelector('button');
// addEventListener 메서드는 동일한 요소에서 발생한 동일한 이벤트에 대해
// 하나 이상의 이벤트 핸들러를 등록할 수 있다.
$button.addEventListener('click', function () {
console.log('[1]button click');
});
$button.addEventListener('click', function () {
console.log('[2]button click');
});
// 이건 하나 이상의 이벤트 핸들러 등록! 등록된 순서대로 호출!
</script>
Tip) addEventListener 메소드는 하나 이상의 이벤트 핸들러를 등록할 수 있다.
단, 참조가 동일한 이벤트 핸들러를 중복 등록하면 하나의 이벤트 핸들러만 등록된다.
<button>Click me!</button>
<script>
// 중복 등록하면 하나의 이벤트 핸들러만 등록
const $button = document.querySelector('button');
const handleClick = () => console.log('button click');
// 참조가 동일한 이벤트 핸들러를 중복 등록하면 하나의 핸들러만 등록된다.
$button.addEventListener('click', handleClick);
$button.addEventListener('click', handleClick);
</script>
이벤트의 종류
마우스 이벤트
이벤트 명 | 이벤트 타입 | 설명 |
click | onclick | 마우스 버튼을 클릭했을 때 |
dbclick | ondbclick | 마우스 버튼을 더블 클릭했을 때 |
mousedown | onmousedown | 마우스 버튼을 눌렀을 때 |
mouseup | onmouseup | 누르고 있던 마우스 버튼을 놓았을 때 |
mousemove | onmousemove | 마우스 커서를 움직였을 때 |
mouseenter | onmouseenter | 마우스커서가 요소안으로 들어오는 순간 발생한다. (* 버블링되지 않는다) |
mouseover | onmouseover | 마우스 커서가 해당 요소 위에 위치할 경우 발생 요소 위에 위치할 때 1회만 발생하며 연속해서 발생하지 않는다. (* 버블링 된다) |
mouseleave | onmouseleave | 마우스 커서를 HTML 요소 밖으로 이동했을 때 (* 버블링되지 않는다.) |
mouseout | onmouseout | 마우스 커서가 해당 요소 위를 벗어 날때 발생 (* 버블링 된다) |
키보드 이벤트
이벤트 명 | 이벤트 타입 | 설명 |
keydown | onkeydown | 키보드(모든 키)를 누를 때 키보드가 눌려서 내려갈 때 1회만 발생한다. |
keypress | onkeypress | 문자 키를 눌렀을 때 연속적으로 발생 (control, option, shift, tab, delete, 방향 키는 발생 x) |
keyup | onkeyup | 누르고 있던 키를 놓았을 때 한번만 발생 |
포커스 이벤트
이벤트 명 | 이벤트 타입 | 설명 |
focus | onfocus | HTML 요소가 포커스를 받았을 때 (* 버블링되지 않는다) |
blur | onblur | HTML 요소가 포커스를 잃었을 때 (* 버블링되지 않는다) |
focusin | onfocusin | HTML 요소가 포커스를 받았을 때 (* 버블링 된다) |
focusout | onfocusout | HTML 요소가 포커스를 잃었을 때 (* 버블링 된다) |
폼 이벤트
이벤트 명 | 이벤트 타입 | 설명 |
submit | onsubmit | form 요소 내의 submit 버튼을 클릭했을 때 |
reset | onreset | form 요소 내의 reset 버튼을 클릭했을 때 (최근에는 사용 x) |
change | onchange | <input>, <textarea> 등 폼 요소 콘텐츠의 내용이 변경 되었을 때 |
select | onselect | <input>, <textarea>등 요소 내의 텍스트의 일부 혹은 전부가 선택 되었을 때 발생한다. |
값 변경 이벤트
이벤트 명 | 이벤트 타입 | 설명 |
input | oninput | input(text, check, radio 등), select, textarea 요소의 값이 입력 되었을 때 |
change | onchange | input(text, check, radio 등), select, textarea 요소의 값이 변경 되었을 때 (change 는 포커스를 잃었을 때 작동한다) |
readystatechange | onreadystatechange | HTML 문서의 로드와 파싱 상태를 나타내는 readyState 프로퍼티 값('loading', 'interactive', 'complete')이 변경 될 때 |
DOM 뮤테이션 이벤트
이벤트 명 | 이벤트 타입 | 설명 |
DOMContentLoaded | onDOMContentLoaded | HTML 문서의 로드와 파싱이 완료되어 DOM 생성이 완료 되었을 때 |
프레임/뷰 이벤트
이벤트 명 | 이벤트 타입 | 설명 |
resize | onresize | 브라우저 윈도우(window)의 크기를 리사이즈할 때 연속적으로 발생 |
scroll | onscroll | 웹페이지 또는 HTML 요소를 스크롤할 때 연속적으로 발생 |
리소스 이벤트
이벤트 명 | 이벤트 타입 | 설명 |
load | onload | DOMContentLoaded 이벤트가 발생한 이후, 모든 리소스(이미지, 폰트등)의 로딩이 완료 되었을 때 발생 |
unload | onunload | 리소스가 언로드될 때(주로 새로운 웹페이지를 요청한 경우) |
abort | onabort | 리소스 로딩이 중단되었을 때 |
error | onerror | 리소스 로딩이 실패했을 때 |
이벤트 발생한 요소객체에 접근하는 3가지 방법
Tip) 개발자 도구로 들어가서 console(콘솔) 확인 하시면 됩니다.
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>이벤트 핸들러</title>
<style>
button.btnTest{
background-color: pink;
border: 1px solid black;
}
</style>
</head>
<body>
<button id="btn1" class="btnTest">기본 이벤트 방식</button>
<button id="btn2" class="btnTest">표준 이벤트 방식</button>
<button class="btnTest" onclick="test(event);">인라인 이벤트 방식</button>
<script>
// 고전(기본) 이벤트 모델 방식
document.querySelector("#btn1").onclick = function(e){
// PointerEvent : 마우스를 시장할 때 발생하는 이벤트
// MouseEvent 로 뜰 때도 있다.
// window.event = e
console.log(e); // PointerEvent 객체 : 이벤트 발생시 매개변수 e로 전달
console.log(window.event) // 이벤트 발생시 내부적으로 전달돰 (매개변수 처럼)
// 현재 이벤트가 발생한 요소객체를 가져오고자 한다면?
// window.event.target == e.target == this
console.log(window.event.target); // target 의 속성값이 : 해당요소
console.log(e.target); // 매개변수의 타겟 속성 : 해당요소
console.log(this); // 현재 요소객체
window.event.target.style.backgroundColor = "pink";
e.target.innerHTML = "버튼클릭됨"
this.style.color = "white"
}
document.querySelector("#btn2").addEventListener("click", function(e){
console.log(e.target); // 고정이벤트 방식과 마찬가지로 사용가능
console.log(window.event.target);
console.log(this)
})
// 인라인 이벤트 모델 방식
function test(e) {
console.log(window.event.target);
console.log(e.target);
console.log(this);
// 위 두 방식은 해당요소 "객체".이벤트호출 this로 매핑된 객체가 요소객체가 됨
// 근데 일반적인 선언적 함수내에서 this호출시 window객체와 매핑됨
}
</script>
</body>
</html>
이벤트 제거하기
방법1 : null 를 대입
이벤트 속성에 null을 대입하면 이벤트 핸들러를 제거할 수 있다
document.querySelector("#btn1").onclick = null; // 이벤트 제거
방법2 : removeEventListener 메서드 사용
단, addEventListener 메소드에 전달한 인수와 removeEventListener 메소드에 전달 한 인수가 일치 하지 않으면 이벤트 핸들러가 제거 되지 않는다.
<button>Click me!</button>
<script>
const $button = document.querySelector('button');
const handleClick = () => console.log('button click');
// 이벤트 핸들러 등록
$button.addEventListener('click', handleClick);
// 이벤트 핸들러 제거
// addEventListener 메서드에 전달한 인수와 removeEventListener 메서드에
// 전달한 인수가 일치하지 않으면 이벤트 핸들러가 제거되지 않는다.
$button.removeEventListener('click', handleClick, true); // 실패
$button.removeEventListener('click', handleClick); // 성공
</script>
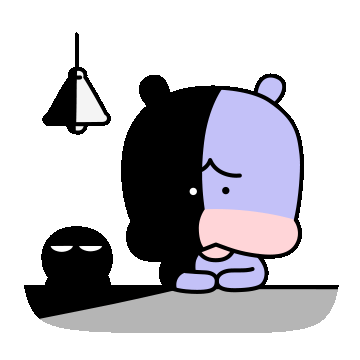
감사합니다.
'프론트엔드' 카테고리의 다른 글
[Front] JS heap, Listener, Node를 신경 쓴 메모리 누수 이야기 (0) | 2024.04.30 |
---|---|
[Front] SSR vs CSR : 웹 애플리케이션 렌더링 방식 비교 (0) | 2024.01.25 |
[Template] Thymeleaf 타임리프 : 백엔드의 렌더링 방법 (0) | 2024.01.09 |
[Front] HTML 요소에 data 속성 사용하기 : 더 깔끔하게 (0) | 2024.01.02 |
[Fornt] 선택자와 요소 : 기본적인 이해 (0) | 2023.11.24 |
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
링크
TAG
- spring
- 오라클
- Spring Security
- 네트워크
- Mac
- 데이터 베이스
- AJAX
- 템플릿
- git
- 개발
- 프로세스
- 개발환경
- DBeaver
- JavaScript
- 개발자
- 프론트
- 비동기
- 개발블로그
- java
- 자바스크립트
- Fetch
- aws
- Front
- 디자인패턴
- Cors
- 코딩테스트
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 | 31 |
글 보관함